A lot of newcomers believe (or are told) that coding is an absolutely essential skill in order to create even your very first game. That’s not true! In fact, we have a lot of engines and tools/ plugins which allow you to create a complete game without having any prior coding knowledge.
It is all done with the magic of “visual scripting” in which you use visual programming blocks instead of textual code. This lets you introduce gameplay elements without having to bother with programming syntax. Well, you still need to apply logical thinking, because “visual scripting” is basically a fancy GUI slapped on top of hidden code.
So, is coding required for game development? It honestly isn’t THE most necessary thing to learn if you’re just starting out and want to create something simple like a puzzle game or 2D platformer. Instead, you can employ a game engine that gives you things like a visual scripting language, scene editor, asset store, graphics rendering engine, etc. and all you have to do is bring your creative vision to reality by applying logic and creating the artwork.
Even artwork and sound effects can be purchased or downloaded for free from various online stores/ public repositories. Just make sure to add your own twist to match the assets with your game theme, it shouldn’t be a total rip-off (i.e. asset flip).
Even though you can simply “drag and drop” modules that add functionality to your game, you need to understand certain basic concepts of coding. Like what a variable is, how objects and methods work, conditions, functions, loops, etc. Understand that all the syntax is being masked by something like BOLT or Playmaker, but underneath all these nodes it is just regular code from whatever language your visual scripting system uses.
Logic + Syntax creates a program, and a plugin will only take care of the second half. You still need to input logic, and knowing basic coding principles will allow you to write more efficient “code” and approach complex problems from new perspectives.
Will You Need To Learn Coding At Some Point?
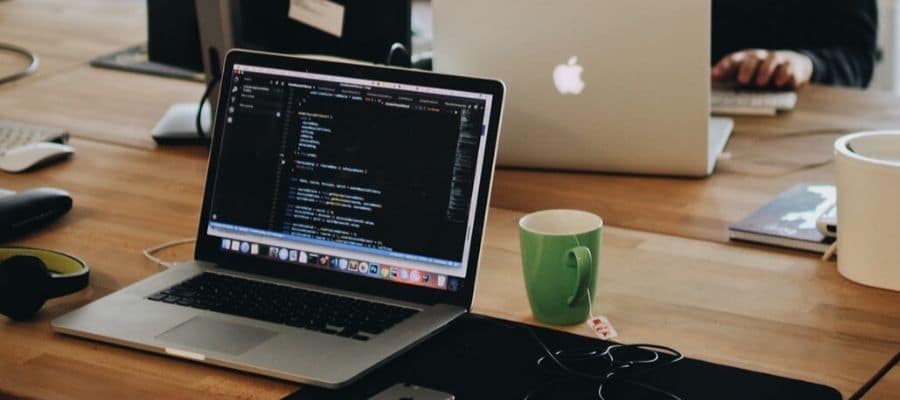
Probably, but only if you’re serious about game development. And even then, the degree to which you must be proficient in coding highly depends on your role within the development team.
Are you a programmer tasked with writing the AI and game logic? In that case, you most certainly need to know at least 2 languages, the most common being C# and C++. A lot of game programmers also learn Java and Python.
If you’re an artist, you probably don’t have to be very good with coding, but a knowledge of the basics will come in handy at some point. As a designer, you may or may not have to provide your input regarding code since your primary task is directing the rest of the team and coming up with ideas for how the game will play instead of writing the game itself.
What Is Visual Scripting?
What comes to your mind when you think of text-based coding? Probably something like this:
#include <iostream>
using namespace std;
int main()
{
cout << "Hello, World!";
return 0;
}
That is a simple “Hello World” program written in C++ code; it displays “Hello World” on the screen. Now as you can see, computers don’t quite understand human languages such as English which is why we have to use special “code” to communicate with them.
For a lot of people who are new to game development, this is a massive barrier. You have to learn how to translate your logical thoughts into code, and you have to be good at it. A visual programming language takes out this intermediate requirement by providing you with visual blocks that represent various things like functions, objects, and variables.
You can link these visual blocks or “nodes” with lines to establish a connection between them, and create stuff like game logic or AI without having to write a single line of code. Here’s an example of a Hello World program using MIT’s Scratch VPL (visual programming language)-
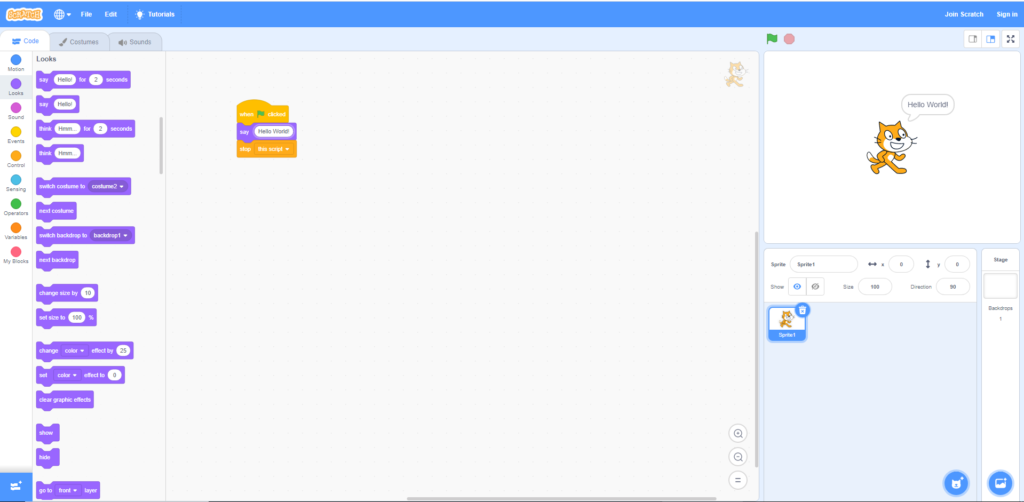
As you can see, we created a little script that displays “Hello World” when you click the green flag. Code is broken down into easy to understand visual blocks, which makes it much easier for novices and beginners to understand. Apart from educational purposes, this has its uses within game studios as well.
Because now artists and designers can make additions or changes to the project much more easily without having any significant coding skills. This will accelerate the development pipeline by making things easier for programmers.
Scratch is just one of the many visual programming languages. Minibloq, Ardublock, and mBlock are some examples of visual programming languages. In the realm of game development, an example of GUI-based programming languages is FlowGraph, which is built into the CryEngine sandbox editor. Unity has its own visual scripting system, as does Unreal Engine (in the form of Blueprints).
Some Great Tools For Creating Games Without Coding
Unity visual scripting:
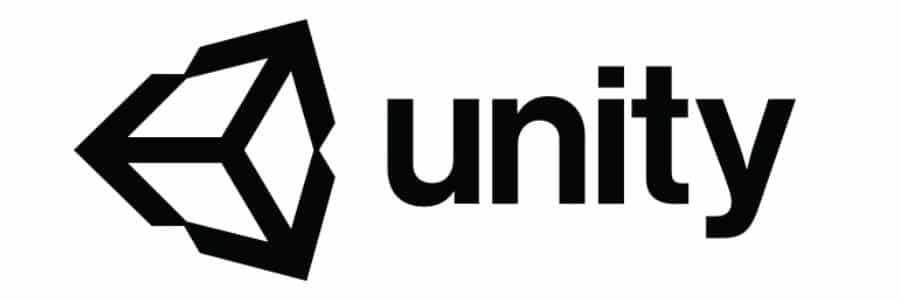
The native visual scripting system for Unity, it’s built right into the editor and requires no additional download. Designed around DOTS (data-oriented technology stack), it is optimized to run multithreaded code for future high-performance games that can take advantage of the latest multi-core CPUs. The Unity Visual Editor sports a clean UI with a blackboard and stacks and generates C# code at runtime to maximize performance.
It also supports live editing in play mode (feature listed on the roadmap), along with notes and color grouping for better organization of code. The support for stacking will reduce noodle-graph spaghetti code that is a major issue with most visual scripting systems.
You also get very reliable and intuitive debugging tools built into the system to work alongside DOTS. And high-performance visual code is almost guaranteed out of the box, thanks to a combination of C# + Burst compiler + optimized memory layout.
BOLT
A visual scripting plugin for the Unity game engine, it lets you implement game mechanics and logic right from the editor without any need for writing code.
AI, Physics, rendering, and much more can be created super fast with BOLT. It is viable for the entirety of a game’s development pipeline, from the prototype stage to the finished product.
GameMaker Studio:
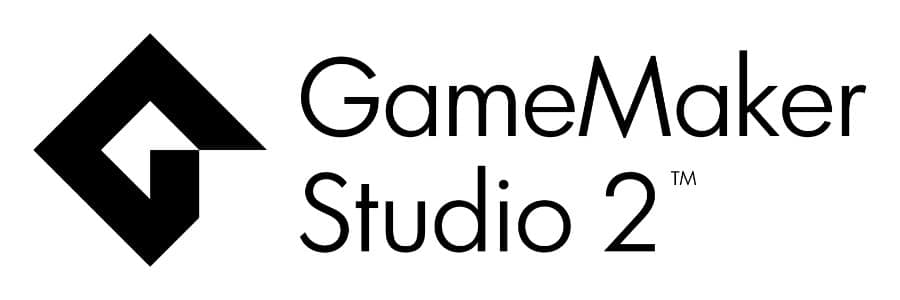
A game engine, it has its own scripting language- GML, which is much easier to learn than C++. GameMaker Studio also supports visual scripting through a simple drag & drop UI geared towards novice coders who can easily whip up simple 2D platformers and puzzle games without much in the way of any actual coding.
It is also used for prototyping purposes by actual game studios. Some really cool indie games like Hotline Miami and Spelunky were made with GameMaker Studio.
Construct 3
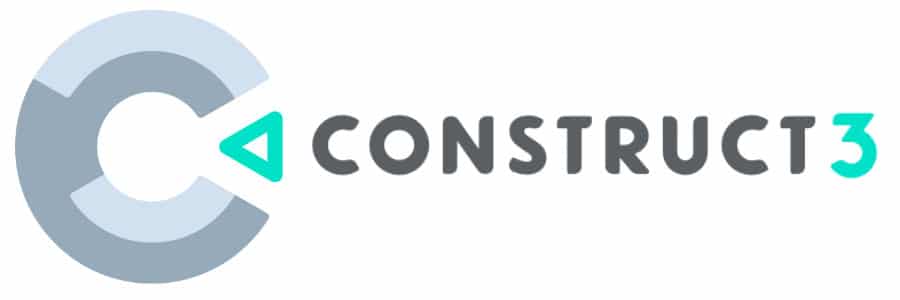
Drag and drop 2D game development toolkit which is primarily used for making HTML5 browser games, prototypes, teaching kids coding basics, etc.
Construct 3 is used to create RTS, platformer, and puzzle games. Construct works on everything from an Android tablet to a Macbook and boasts super-fast performance.
Unreal Engine
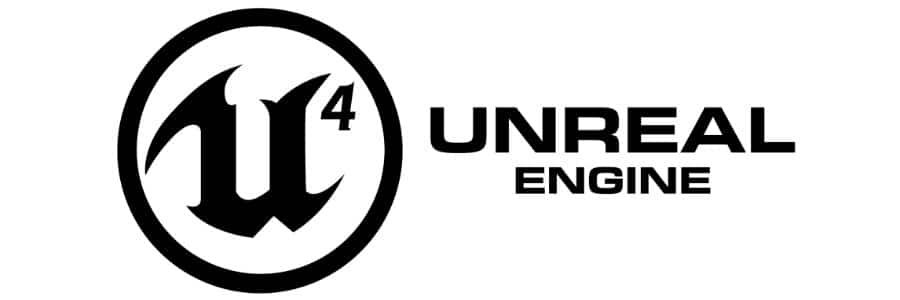
Unreal has had it for a while in the form of Blueprints. It uses a node-based interface and can be accessed within the UE editor. Blueprints are used to define object-oriented classes or objects and are extremely versatile.
It lets developers access nearly all concepts and techniques that are traditionally available only to those who use text-based programming.
Basics of Visual Scripting With BOLT
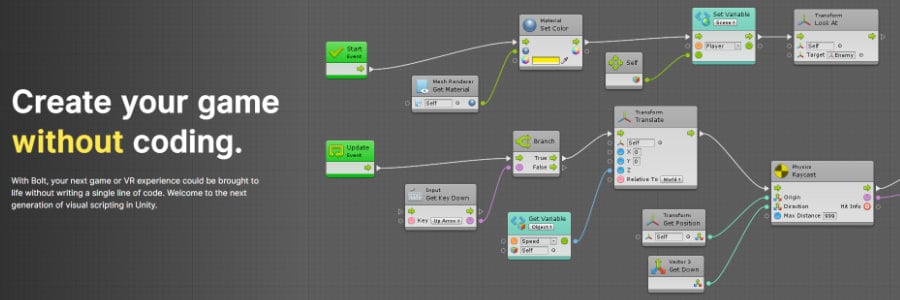
We know that Unity is currently working on its native visual scripting system. Still, if you want a reliable and well- documented plugin for visual scripting with tons of tutorials out there, Bolt is the answer.
Plenty of “real coders” out there will complain that visual programming isn’t as customizable, that it’s not very scalable. Any while they may be right, most of them miss the point on why we use it.
It’s for both novices as well as experienced coders who want to focus on just the game logic, by distancing themselves from the syntax and leaving that up to the plugin or engine.
But you’ll still have to understand the underlying mechanics, regardless of whether you choose to write your own code or drag around icons in the editor. Well, first things first- install Bolt. Starting out, you should stick with Human Naming. It is better for novices and makes the task of finding nodes super easy.
Then, you need to add a component called the Flow Machine. Flow machines take place within Macros, which are basically like a script to control everything within your game. Use traditional macros, not the embed type. Upon creating the flow machine, an object variable gets added. Now multiple macros attached to this object can use the same variables – very similar to public variables in programming.
A variable is simply a name tied to a storage location in memory that stores unknown or known information of a certain type that can vary over time (not constant). An integer variable called int can store integers like 3, 100, 55, etc. A character variable like char can store characters such as a, b, x, etc. Constants are the opposite of variables since they have a fixed value which cannot change.
Back to scripting with Bolt- once you’ve created the flow graph and macro, you can start editing the graph. There are plenty of variable types, but the 3 most important ones are- Graph, Object, and Scene. Graph variables are private and can only be accessed by the current specific graph. T
his is what you’d use to set the default max health of a character or to “hard code” a certain time value. Object variables are accessible by any macro and are used to access the animator attached to another object or for adding variables to assign specific audio clips from the project panel. Scene variables are global variables accessible by any object within a certain scene.
Next, you’ve got the Start and Update nodes. The start is called only once when the game starts. Use it to set starting HP and gold values, reference a certain starting position, etc.
The update is called several times each second and is constantly checking for changes in the system. You can use it to check for a change in player input, or for updating the health bar over time.
Unity’s Very Own Visual Scripting System
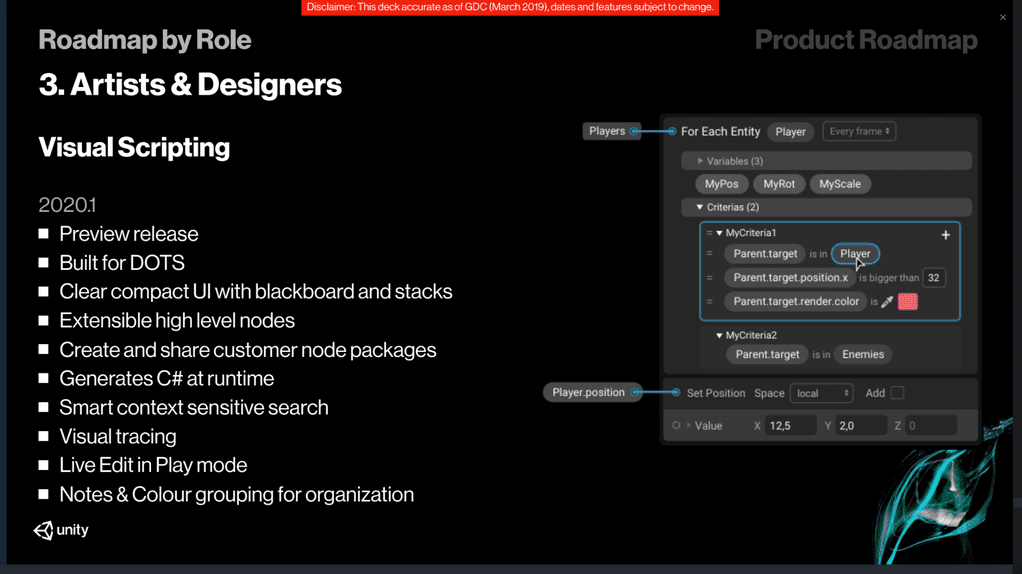
A lot of Unity users had been asking for a visual scripting system for a long time. And their wish was finally granted last year with the official announcement of visual scripting support during the 2019 GDC conference.
It has been implemented in a Beta state into the engine since version 2019.2, and the stable release will take place with 2020.1 during Spring this year which is going to add new features along with better performance. Until now, if you wanted visual scripting within Unity, your only options were plugins such as Bolt or Playmaker.
But with official support, Unity’s own VSL is guaranteed to gain popularity over 3rd party tools since it’s going to receive more frequent updates with (possibly) better features. It is accessible to beginners, as well as programmers since you can write your very own nodes or code blocks. Unity’s visual scripting language will be used in conjunction with the DOTS animation system and will feature a bunch of cool stuff like Live Edit in Play mode.
And it performs pretty well since the underlying code generated by this visual language is in C#. Check out some of the features they outlined for their visual scripting language in the roadmap, some of these can already be experienced while others will arrive in the full 2020.1 release
Does all this mean that BOLT is now dead? Well, at the moment it’s still very much alive and a lot of people using Unity have trained with Bolt. Not to worry, because all your skills will carry over to Unity’s own VSL if you choose to switch over.
Generally, when a company introduces something like this, a feature previously only found in 3rd party plugins, said 3rd party plugin falls out of favor. Unity’s own VSL will be created by people who know and understand the engine at a core level, better than anybody else.
It is supposed to perform faster, and have fewer bugs. It will also be updated quicker, and receive new features at a faster rate compared to BOLT since there is a much larger team working on it. And, it will be free (although you can get the “non-profit” version of Bolt for free if you’re a student or Youtuber).
The one thing that Bolt has going for it, is the non-ECS nature. ECS or Entity Component System is at the core of Unity’s DOTS (Data Oriented Tech Stack). Read more about it here.
If it sounds confusing, the basic difference is that Unity’s visual scripting system will favor data-oriented design whereas Bolt relies on traditional OOP (Object Oriented Programming). This is all part of Unity’s shift towards multithreaded code which is part of their vision for the future.
Conclusion
For larger projects, you’re better off writing your own code instead of using visual scripting systems like Bolt. You’ll need to learn variables, logic, loops, conditions, etc. anyways since the system only hides the Syntax, it doesn’t actually create the logic.
lus, debugging is way easier with text-based code, since you can change and expand stuff much more easily. Visual code is easier to start with but hits a ceiling pretty fast.